Effective Strategies for Mastering React Design Patterns
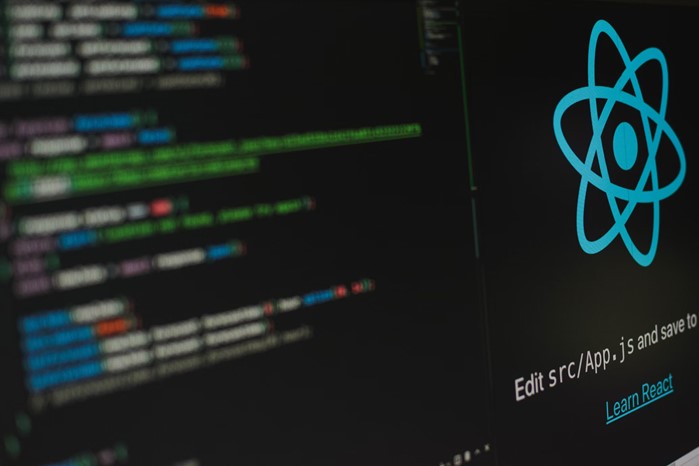
One of the most widely used tools for creating websites and apps is React. Although it is strong and adaptable, managing your app may get more challenging as it expands. Your code will be easier to read, update, and fix if it is organized using React design patterns. Let’s discuss some simple techniques to help you become proficient with React design patterns.
What Are React Design Patterns?
React design patterns are simple solutions to common problems in front-end development services. They help developers organize and structure their code so it’s easier to manage and maintain. In React, design patterns are especially helpful because they guide how to handle state, organize components, and manage data throughout your app.
React design patterns help in improving the organization, effectiveness, and readability of your code. They offer answers to typical problems including handling complicated states, guaranteeing efficient data flow, and logically arranging components. Developers may create scalable, clean apps that are simpler to update and maintain over time by adhering to these conventions.
Why Should You Learn React Design Patterns?
Learning design patterns helps in several ways:
- Cleaner Code: You’ll write code that’s easy to read and understand.
- Fewer Mistakes: Patterns guide you, so you’re less likely to make the same mistakes over and over.
- Easier to Scale: As your app grows, these patterns help keep everything organized and running smoothly.
In short, design patterns make your life as a developer easier and your app better.
Start with the Basics
Before jumping into advanced React design patterns, make sure you understand the basics of React. If you’re not comfortable with things like components, props (properties), and state (data that can change), it’s best to learn those first. Once you’ve got a good grip on these basic ideas, you’ll find design patterns much easier to pick up.
Use Simple Patterns First
When you’re new to React, it’s a good idea to start with simple patterns. These are easy to understand and will help you get the hang of things. You’ll lay a strong foundation by first learning the fundamentals. Later on, it will be simpler to deal with more complex ideas because of this.
Don’t worry about using complicated solutions right away. Focus on managing the state and organizing components first. Once you’re comfortable with these, you can try more complex React design patterns. Taking it step by step will make the process smoother and help you become more confident as you go. Keep it simple at the start, and you’ll be better prepared for more challenges down the road.
Manage Your State Carefully
Carefully managing your state is the key to developing a React application that is both user-friendly and efficient. The state React component pattern helps your program react to user activities and manages its data. Effective state management makes it simpler to update and maintain your application.
Having too many states or putting them in the incorrect components is a typical error that can make your code jumbled and challenging to handle. Instead, keep your state simple and place it in the right components. Use React’s hooks like useState for local state management and useContext for sharing state between components. By managing your state properly, you ensure your app runs smoothly and is easy to scale as it grows.
Focus on Reusability
One of the great things about React is the ability to reuse your components. A component is just a piece of your app, like a button or a navigation menu. If you can build small, reusable components, you’ll save time and keep your code clean.
Think of React component patterns as building blocks. The more reusable they are, the easier it is to build and change things in your app. For example, you can make a button component that works anywhere in your app. If you ever need to change the style or behavior of buttons, you can just update the button component, and the change will happen everywhere it’s used.
Use React Hooks
Managing state and other React.js design patterns in functional components become easy and powerful with React Hooks. Without using class components, they let you develop code that is clearer and easier to read:
- UseState is one of the most used hooks for managing state inside function components.
- UseEffect is another crucial hook that allows you to handle side effects, such as etching data or modifying the DOM, following the rendering of your component.
- Another helpful hook is useContext, which makes it simple to share data between many components without having to manually pass props down.
As your React application expands, you may use hooks to make your code adaptable, comprehensible, and maintainable.
Keep Components Small and Simple
A common mistake is making components too big. When a component tries to do too much, it becomes difficult to manage and test. The best approach is to break your app into smaller, focused components that each handle one task.
For instance, divide a huge component into two distinct components: one for managing user input and another for displaying the material, rather than having one large component that does both tasks. This simplifies updating and following your code. It is also easier to test smaller components. Before assembling your project, you may make sure that every component functions as intended.
The key to mastering React design patterns and best practices is figuring out the best approach to structure and arrange your application. Start with the fundamentals, keep things easy, and then progressively experiment with more complex React.js design patterns as you become more at ease. You can create scalable, effective, and clean React apps with practice. You will soon be able to handle any problem if you continue to experiment!